[LeetCode][python3]0006. ZigZag Conversion
Start the Journey
N2I -2020.03.15
1. My first try
class Solution: def convert(self, s: str, numRows: int) -> str: gap=(numRows-1)*2 if len(s)<2 or gap<=0: return s ans=[] for i in range(numRows): if i==0 or i==numRows-1: ans+=s[i::gap] #print(ans) else: dic={} index=0 for item in s[i::gap]: dic[index]=item index+=2 index=1 for item in s[(gap-i)::gap]: dic[index]=item index+=2 for index in range(len(dic)): ans.append(dic[index]) #print(ans) return ''.join(ans)
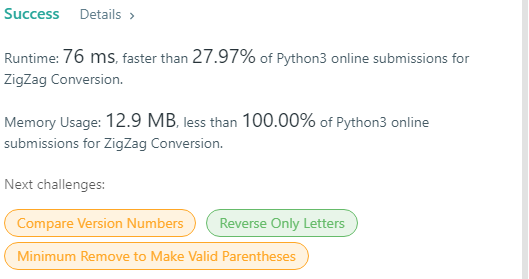
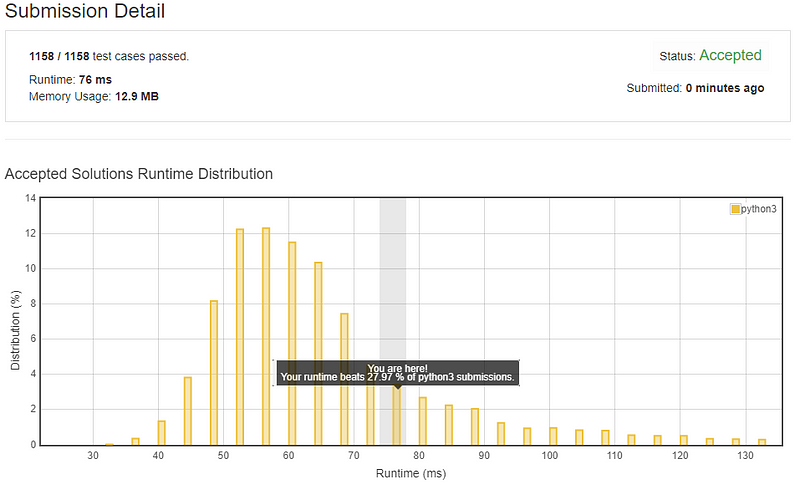
Explanation:
Just another slow solution.
2. A better solution
class Solution: def convert(self, s: str, numRows: int) -> str: #n strings and add them together listy = [''] * numRows sign, counter = 1, 0 if numRows == 1: return s else: for i in s: listy[counter] += i counter = counter + sign if counter == numRows - 1: sign = -1 if counter == 0: sign = 1 finalStr = ''.join(listy) return finalStr
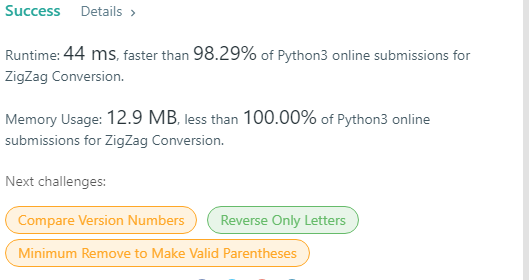

Explanation:
The solution use a
counter
to decide which row the i
char will be thrown into. And throw all char in string by sequence. It is more simple and faster.
Comments
Post a Comment