[LeetCode][python3]0012. Integer to Roman
Start the Journey
N2I -2020.03.17
1. A clean solution
class Solution: def intToRoman(self, num: int) -> str: rom = ["M","CM","D","CD","C","XC","L","XL","X","IX","V","IV","I"] digit = [1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1] result = "" for d, r in zip(digit, rom): result += r * (num // d) num %= d return result

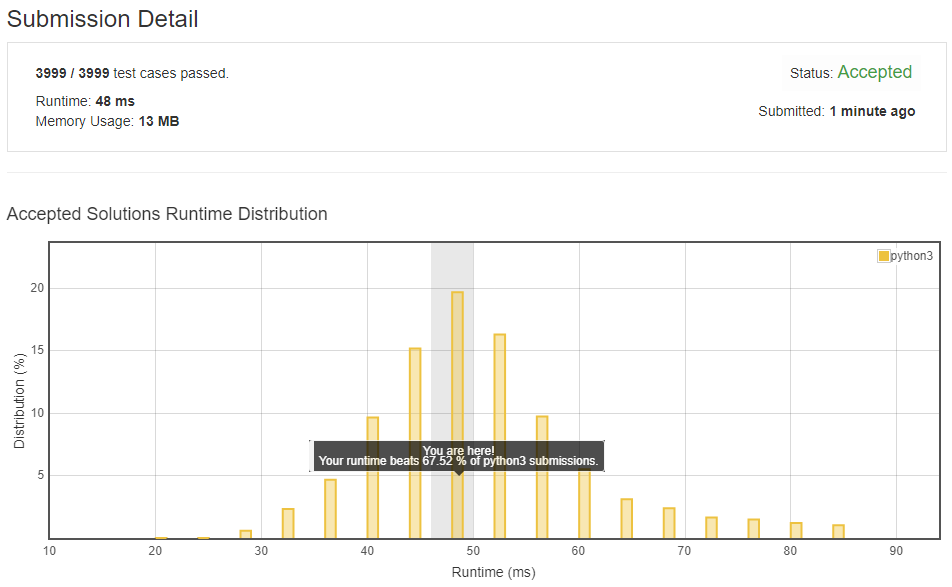
Note:
zip(a,b)
The functionzip
will zip two array items into a two tuple array. the zip array will stop when it reachesmin(len(a),len(b))
print("example of zip(a,b)") a=[1,2,3,4,5] b='abcdef' for n in zip(a,b): print(n) >>>(1, 'a') >>>(2, 'b') >>>(3, 'c') >>>(4, 'd') >>>(5, 'e')
2. My first try
class Solution: def intToRoman(self, num: int) -> str: M=num//1000 num=num%1000 D=num//500 num=num%500 C=num//100 num=num%100 L=num//50 num=num%50 X=num//10 num=num%10 V=num//5 I=num%5 s="" if I==4: if V==0: s="IV" else: s="IX" V-=1 else: s="I"*I s="V"*V+s if X==4: if L==0: s="XL"+s else: s="XC"+s L-=1 else: s="X"*X+s s="L"*L+s if C==4: if D==0: s="CD"+s else: s="CM"+s D-=1 else: s="C"*C+s s="D"*D+s s="M"*M+s return s

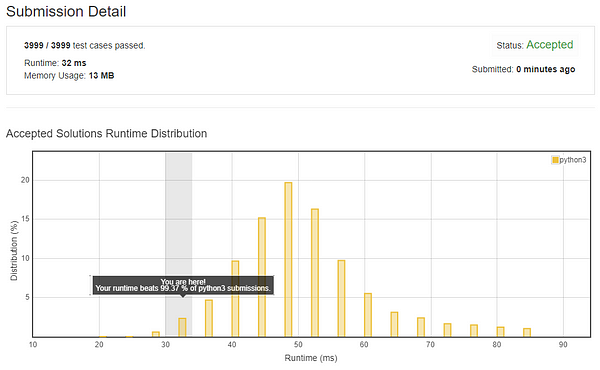
Explanation:
A normal way to solve this problem. It is a bit faster but a longer code. I check out the special cases only when
I=4,X=4,C=4
.
Comments
Post a Comment