[LeetCode][python3]0013. Roman to Integer
Start the Journey
N2I -2020.03.18
1. My first Solution
class Solution: def romanToInt(self, s: str) -> int: rom=["M","D","C","L","X","V","I"] digit=[1000,500,100,50,10,5,1] result=0 if s.find("CM")>=0 or s.find("CD")>=0: result-=200 if s.find("XC")>=0 or s.find("XL")>=0: result-=20 if s.find("IX")>=0 or s.find("IV")>=0: result-=2 for c in s: for r,d in zip(rom,digit): if c==r: result+=d return result
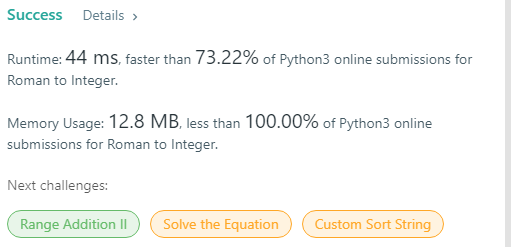
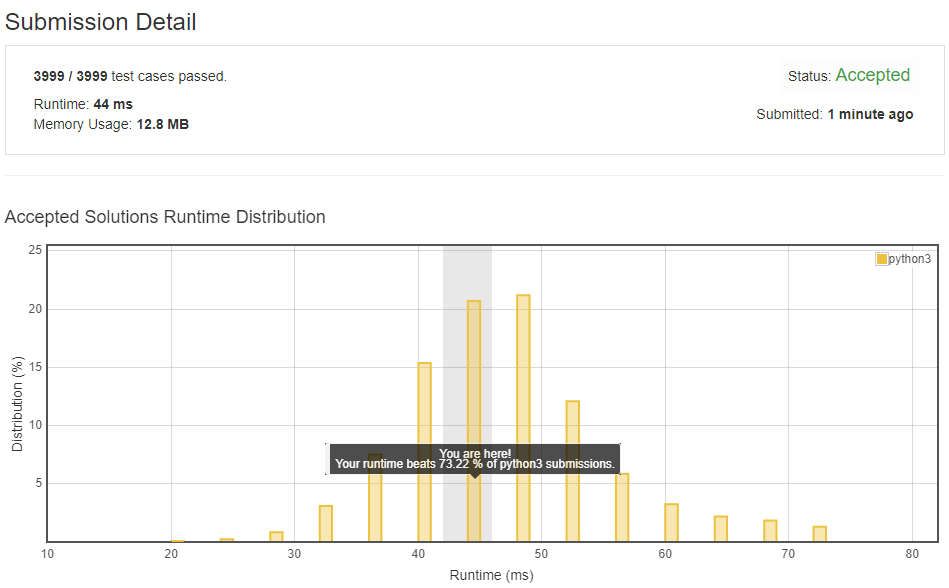
Explanation:
Using
find()
function to solve the problem. When C+M=1000 and CM=900
, this makes the result have a 200 gap. So we minus it first then count it as the same way. Same as CD,XC,XL,IX,IV
.2. Other solution
class Solution: def romanToInt(self, s: str): symbol={'I':1,'V':5,'X':10, 'L':50, 'C':100, 'D':500, 'M':1000} output=[0] for i in s: if symbol[i]>output[-1]: output[-1]=symbol[i]-output[-1] else: output.append(symbol[i]) return sum(output)

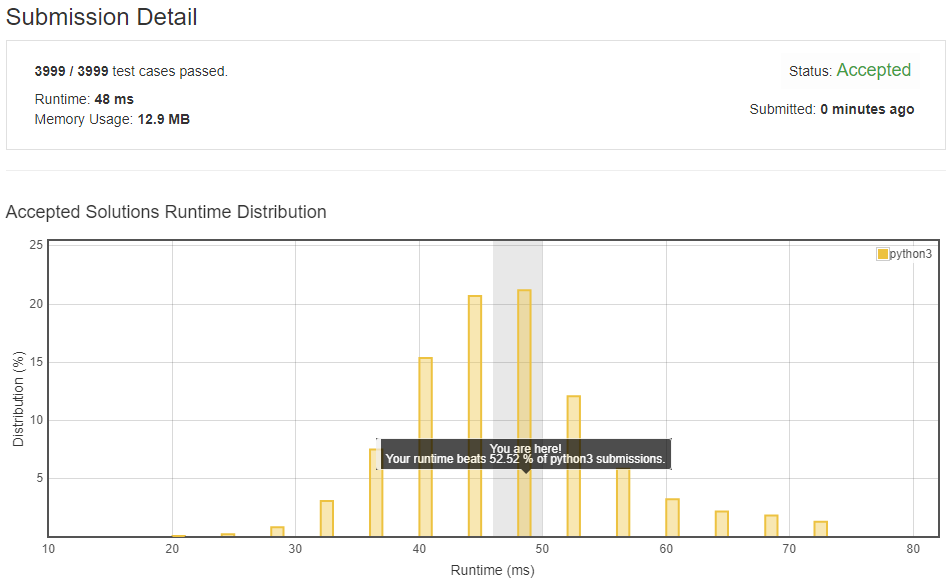
Explanation:
The solution is using
dict
to save the pattern, and check out the special cases when smaller symbol is in front of a bigger symbol.
Comments
Post a Comment