[LeetCode][python3]0020. Valid Parentheses
Start the Journey
N2I -2020.04.02
1. My first solution
class Solution: def isValid(self, s: str) -> bool: upper=['s'] for item in s: if item in ['(','[','{']: upper.append(item) if item==')': if upper.pop(-1)!='(': return False if item==']': if upper.pop(-1)!='[': return False if item=='}': if upper.pop(-1)!='{': return False if upper==['s']: return True return False
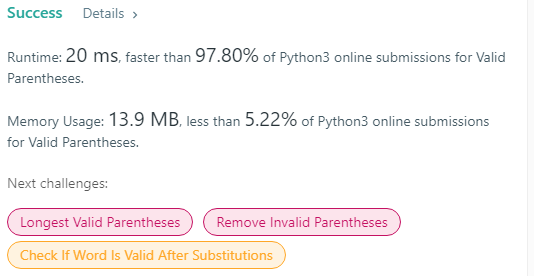
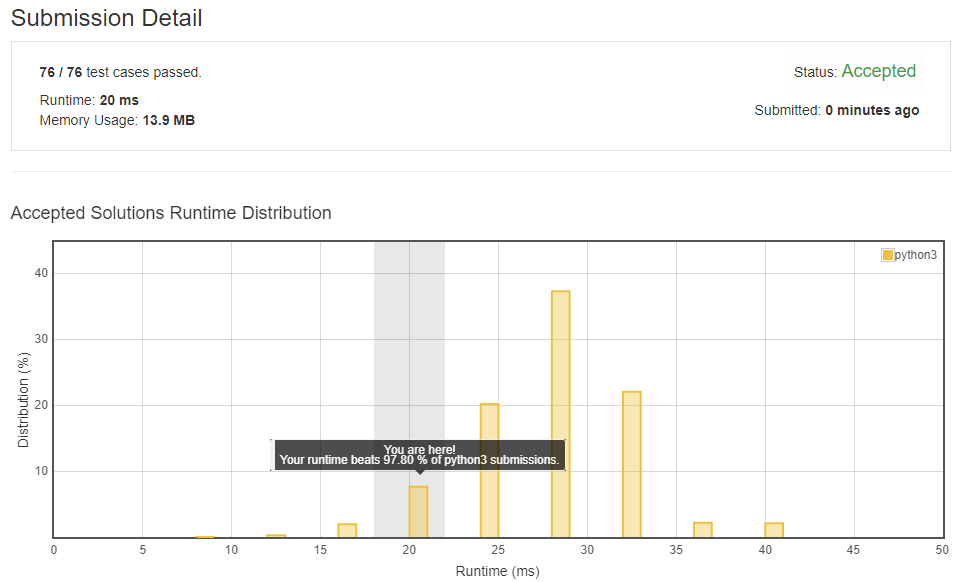
Explanation:
The solution use a buffer to solve this problem. For the elements
['(','[','{']
, has to be matched in the sequence “Last in, First out”. And the element ['s']
to check if buffer is empty.2. A clean solution
class Solution: def isValid(self, s: str) -> bool: mapping = {')':'(', '}':'{', ']':'['} stack = [] if not s: return True for ele in s: if ele in mapping: if stack: top = stack.pop() else: top = '$' if top != mapping[ele]: return False else: stack.append(ele) return not stack
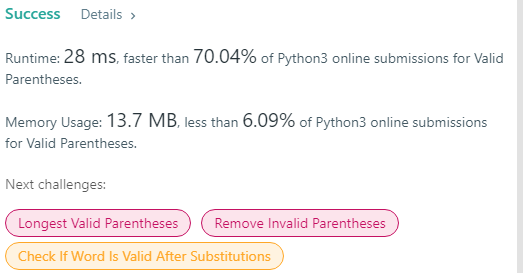
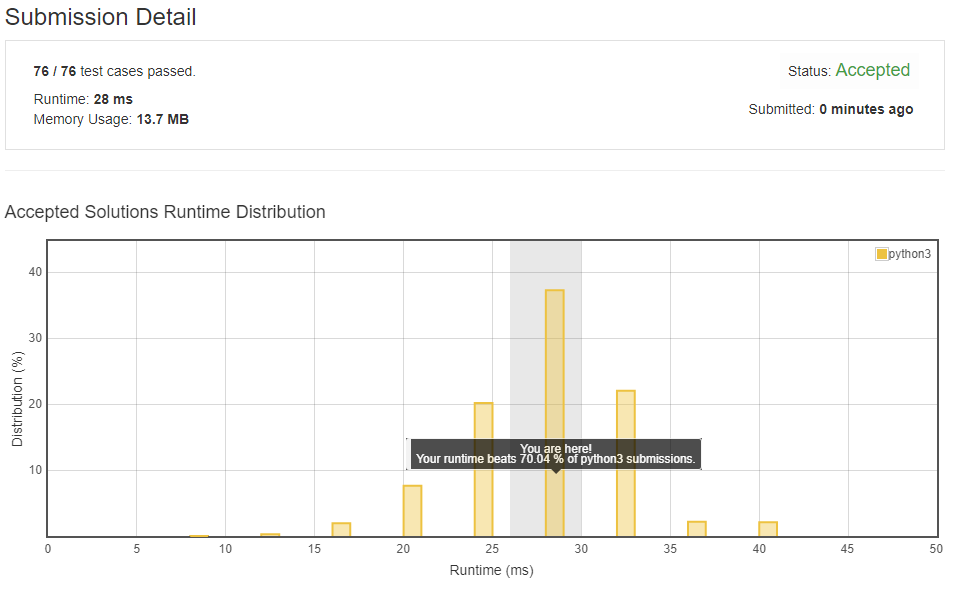
Explanation:
A more flexible way to code the script.
Comments
Post a Comment