[Leetcode][python3]Day01.Single Number (30-Day LeetCoding Challenge)
30 days! Lets go Lets go!
N2I -2020.04.01
1. My Solution
class Solution: def singleNumber(self, nums) -> int: dic={} for i in nums: if i in dic: del dic[i] else: dic[i]=1 for res,tmp in dic.items(): return res

2. A better Solution
class Solution: def singleNumber(self, nums: List[int]) -> int: count = 0 for num in nums: count ^= num print(count) return count
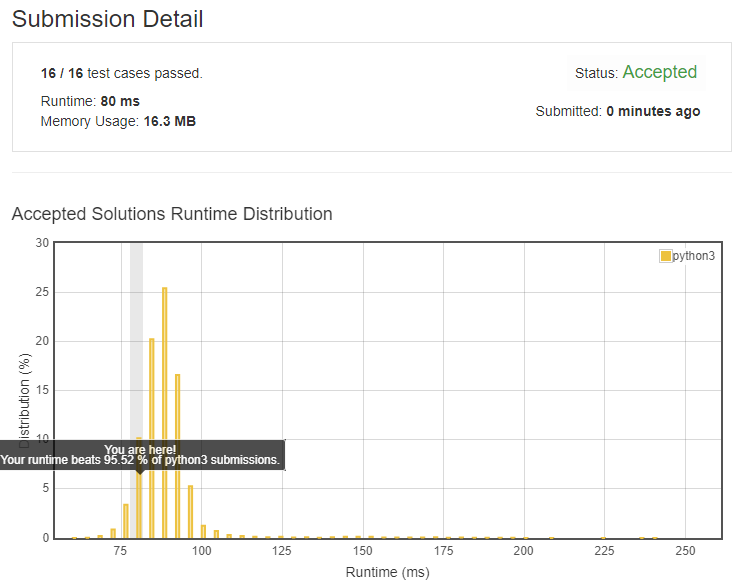
Comments
Post a Comment